Pop ups
In our game we use space to pick up objects. To teach the player the controls we decided to have small prompts pop up when the player approaches something grabbable. After the player has picked up the object once, the prompt will no longer appear.

The red circle in the image is a trigger collider, which spawns the prompt window as a game object when triggered. But having the prompt just appear out of nowhere doesn’t look very good without a transition…
Introducing Interpolation
My idea was to slide the scale of the prompt up and down for the transitions. When the pop up appears, the scale will start at 0 and gradually increase to full size. If we look at the transition as a timeline going from 0 to 1, the size over time could look like this graph.

Having the prompt pop in like this would look much better than no transition at all! However, it looks kind of stale. The velocity of the scale is constant during the transition. There is no feeling, no drama!
Juicy graphs
To make the transition look good, we need to spice up this graph! Grapefrukt did a good talk on juice in video games, where they use a bunch of interpolation techniques to make the game feel juicer.
Naturally I made a static class with some interpolation methods so I can harness this great power.
I wanted the prompt to be a little bouncy, so I combined linear interpolation with a strangely squished Sine graph to create something beautiful:
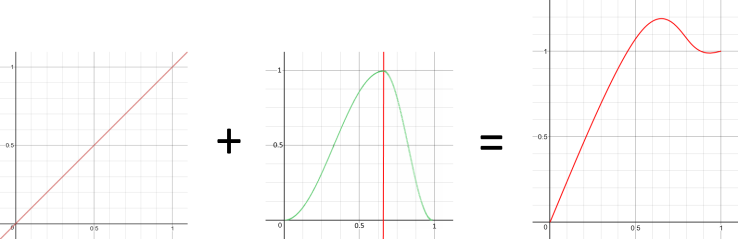
The resulting graph overshoots the target, making the interpolation bouncy! This is the resulting animation in-game:
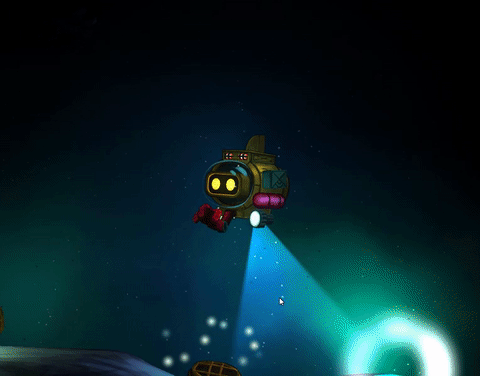
The length of the pop-in and pop-out is identical, but since the pop-out uses a cubic interpolation, the effect is completely different.
Graph heaven
As you can see, I combined two graphs to create a juicy graph. But this is hard-coded for this specific use; the method is called ”Overshoot()” or something. What we actually want is a general way to combine interpolation methods, which is something I would like to do. Also, there is no way of actually seeing or editing the graph visually in the editor.
Turns out there is already a way of doing exactly this and it’s built-in to Unity. RIP hard work. Just goes to show how valuable it is to do a quick search before reinventing the wheel like this (even though I wasted less than an hour).
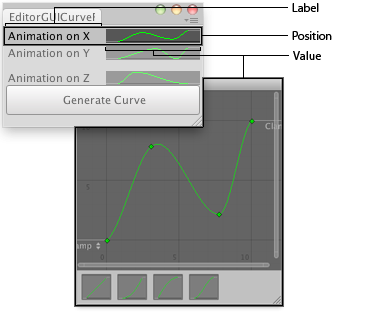
You can take a look at my code here.